SonarQube is an open-source platform for continuously inspecting code quality. It performs automatic reviews with static code analysis to detect bugs, code smells, and security vulnerabilities in your coding projects. SonarQube supports multiple programming languages, including .NET, Java, JavaScript, C++, Python, and more. It integrates with DevOps tools and workflows, making it a versatile choice for improving code quality in software development projects. In this guide, I will show you how you might use SonarQube in a CI/CD process to scan your project for quality issues.
Why Use SonarQube for Scanning .NET Projects?
Code Quality and Maintainability: SonarQube provides detailed reports on duplicated code, coding standards, unit tests, code coverage, code complexities, and technical debt. It helps developers improve code maintainability by identifying problematic areas that need refactoring.
Security Vulnerabilities: With increasing concerns about software security, SonarQube plays a crucial role in identifying and fixing security flaws early in development. It checks the code against a comprehensive database of known vulnerabilities and guides how to fix them.
Automated Code Review: Integrating SonarQube into the CI/CD pipeline allows for automated code reviews, making it easier to spot defects before they make it to production. This automated process saves time during code reviews and helps maintain a high standard of code quality.
Continuous Integration Support: SonarQube integrates seamlessly with various integration tools like Jenkins, TeamCity, and Azure DevOps. This integration ensures that every check-in is scanned, helping to catch issues early and reduce the feedback loop for developers.
Customizable Rules: SonarQube comes with a default set of rules, but it also allows teams to customize the rules based on their coding standards and requirements. This flexibility ensures that the analysis aligns with specific project needs.
Enhanced Team Collaboration: By providing a clear and detailed code quality analysis, SonarQube helps foster better collaboration among team members. Developers can view the issues, understand the reasons behind them, and collectively improve the quality of their codebase.
Regulatory Compliance: Many organisations are required to comply with industry standards and regulations. SonarQube helps ensure that code complies with standards like ISO 27001, SOC 2, and others by enforcing coding rules that align with these regulations.
To follow the rest of the article, you need a working SonarQube installation. If you do not already have one, you can use one of our cloud images below to spin up a production-ready installation.
Step 1: Install .NET SDK
If you have not already done so, we shall begin by installing .Net SDK for our project. The following instructions assume you are working on Ubuntu 20.04.
$ wget https://packages.microsoft.com/config/ubuntu/20.04/packages-microsoft-prod.deb -O packages-microsoft-prod.deb
$ sudo dpkg -i packages-microsoft-prod.deb
$ sudo apt-get update && sudo apt-get install -y dotnet-sdk-8.0
To test your installation, you should be able to run “donet –info”
$ dotnet --info
.NET SDK:
Version: 8.0.204
Commit: c338c7548c
Workload version: 8.0.200-manifests.9f663350
.NET SDKs installed:
8.0.204 [/usr/share/dotnet/sdk]
.NET runtimes installed:
Microsoft.AspNetCore.App 8.0.4 [/usr/share/dotnet/shared/Microsoft.AspNetCore.App]
Microsoft.NETCore.App 8.0.4 [/usr/share/dotnet/shared/Microsoft.NETCore.App]
<text clipped>
Step 2: Install dotnet-sonarscanner tool.
Install the tool using the command below. At a later stage, it can be integrated into our CI process.
$ dotnet tool install --global dotnet-sonarscanner
Sample Code
In order to test the SonarQube vulnerability detection feature, we shall use the code below, which is intentionally vulnerable. Save this file in your .Net project folder as “Database.cs”.
public class SecurityRisks
{
public void ConnectToDatabase()
{
string username = "admin";
string password = "12345"; // Hardcoded password
string connectionString = $"Server=myServerAddress;Database=myDataBase;User Id={username};Password={password};";
// Database connection logic
}
}
As you can see, we have hardcoded database credentials within our code. We put the security of our application at risk when we submit this code into version control or simply share it with another developer. But with SonarQube, we will be able to catch this before we expose our credentials.
Step 3: Begin SonarQube Analysis
We submit the code to SonarQube for analysis using the tool we downloaded earlier. To do this, we need the URL of the SonarQube installation and the login token. To generate a login token for Sonarscanner, login to SonarQube and browse to Administration > Security > Users. Select the user account you will use to run scans and generate a token.
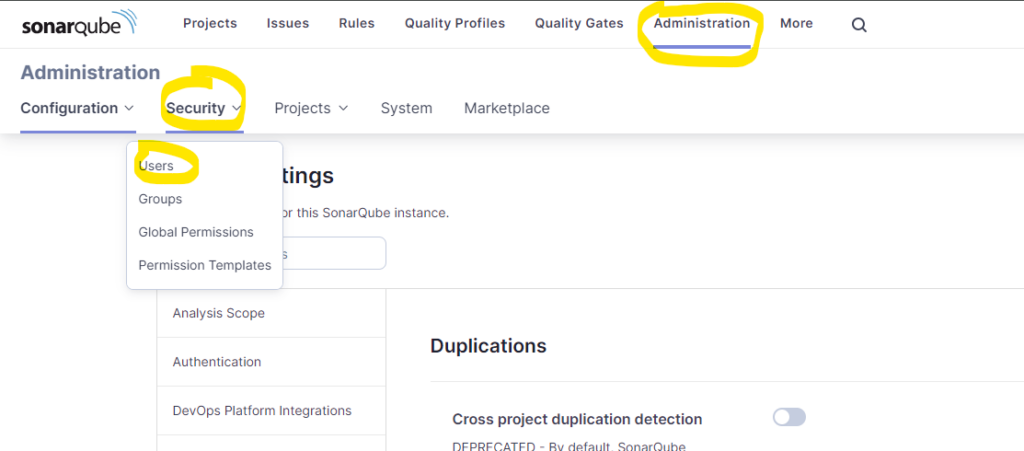
Now that we have the token, we are ready to run dotnet-scanner. The command to use within our project folder is below;
$ dotnet-sonarscanner begin /k:"Project-Name" /d:sonar.host.url="http://1.2.3.4:9000" /d:sonar.login="your-authentication-token"
- Replace
"Project-Name"
with a unique key for your project. It will be created if the project does not already exist in SonarQube. - Replace
"your-authentication-token"
with the token from SonarQube, as shown above.
Now, let’s build the project so that SonarScanner can analyze it.
$ dotnet build
Finally, we can send the analysis results to the server.
dotnet sonarscanner end /d:sonar.login="your-authentication-token"
Step 4: Review the results.
After the analysis is completed:
- Go back to your SonarQube dashboard. Hint: http://1.2.3.4:9000
- Open the project using the “
project-name
” you defined. - Review the issues, code smells, and security vulnerabilities identified by SonarQube. You should see results similar to the screenshot below;
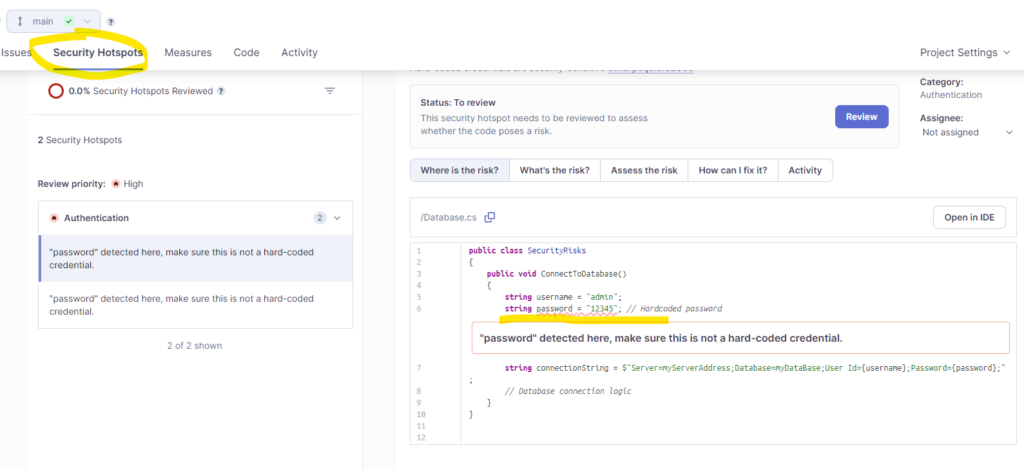
Solution:
To correct this, replace the code in your “Database.cs” file with the code below and then rerun the steps in Step 3.
We now pick the database credentials from the system’s environment in the new code.
# Corrected Code
public class SecurityRisks
{
public void ConnectToDatabase()
{
// Assume environment variables are set for username and password
string username = Environment.GetEnvironmentVariable("DB_USERNAME");
string password = Environment.GetEnvironmentVariable("DB_PASSWORD");
string connectionString = $"Server=myServerAddress;Database=myDataBase;User Id={username};Password={password};";
// Database connection logic
}
}
Additional Tips
- Make sure your project builds correctly before running the SonarScanner to ensure all code paths are analyzed.
- You can customize the analysis by adding a
SonarQube.Analysis.xml
file to your project or by specifying additional parameters during thebegin
command. - Consider integrating SonarQube scanning into your CI/CD pipeline for automated analysis on every build.
Following these steps, you can successfully add and scan your .NET project in SonarQube. This can help you significantly improve the quality and security of your codebase.
Conclusion
Using SonarQube to scan .NET projects significantly enhances the quality of the software, ensures security standards are met, and aids in maintaining a clean and efficient codebase. In this article, we looked at how SonarQube could scan a .Net project for Security Vulnerabilities.
Do you still need help?
Look, our Tech Support Staff live and breathe Cloud Engineering. Let them handle the details, so you can focus on the big picture.
Contact Support